Recommended Posts
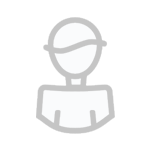
Este tópico está impedido de receber novos posts.
Entre para seguir isso
Seguidores
0
-
Quem Está Navegando 0 membros estão online
Nenhum usuário registrado visualizando esta página.